Map Arlington 4: Adding a JavaScript Front End with Leaflet¶
A Client-side JavaScript UI¶
The last piece of the technology stack I need to add is a user interface that will allow users to interact easily with the maps representing the geospatial data I have stored in PostGIS and rendered with Mapnick and TileStache.
JavaScript is the tool of choice for this, and I will use the Leaflet JavaScript library, so the application stack now looks like this:
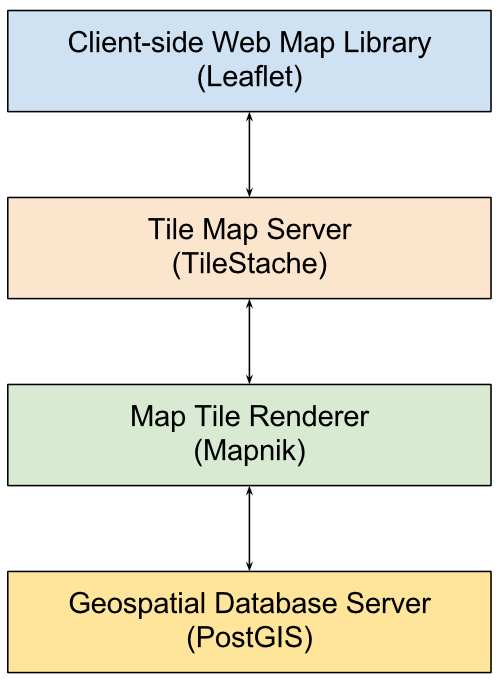
Leaflet makes it easy to enable users to interact with the map server. Here is a first experiment I did to use Leaflet and a public tile server to display a map of the area around where I live:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Leaflet Map 1</title>
<style type="text/css">
@import url(http://cdn.leafletjs.com/leaflet/v0.7.7/leaflet.css);
h1 {
text-align: center;
}
#map {
height: 800px;
width: 800px;
margin-left: auto;
margin-right: auto;
}
footer {
text-align: center;
margin-top: 30px;
}
footer a, footer a:visited {
text-decoration: none;
color: #333;
}
</style>
<script src="http://cdn.leafletjs.com/leaflet/v0.7.7/leaflet.js"></script>
</head>
<body>
<h1>Leaflet Map 1: 38.8605579, -77.1166921</h1>
<div id="map"></div>
<script>
var map = L.map('map').setView([38.8605579, -77.1166921], 15);
L.tileLayer('http://otile{s}.mqcdn.com/tiles/1.0.0/map/{z}/{x}/{y}.jpg', {
attribution: 'Portions Courtesy NASA/JPL-Caltech and U.S. Depart. of Agriculture, Farm Service Agency. Tiles Courtesy of <a href="http://www.mapquest.com/" target="_blank">MapQuest</a> <img src="http://developer.mapquest.com/content/osm/mq_logo.png">',
maxZoom: 19,
subdomains: '1234'
}).addTo(map);
</script>
<footer>
<a href="http://validator.w3.org/check/referer">
<strong> HTML </strong> Valid! </a>
</footer>
</body>
</html>
Rendered in a web browser, it looks like this:
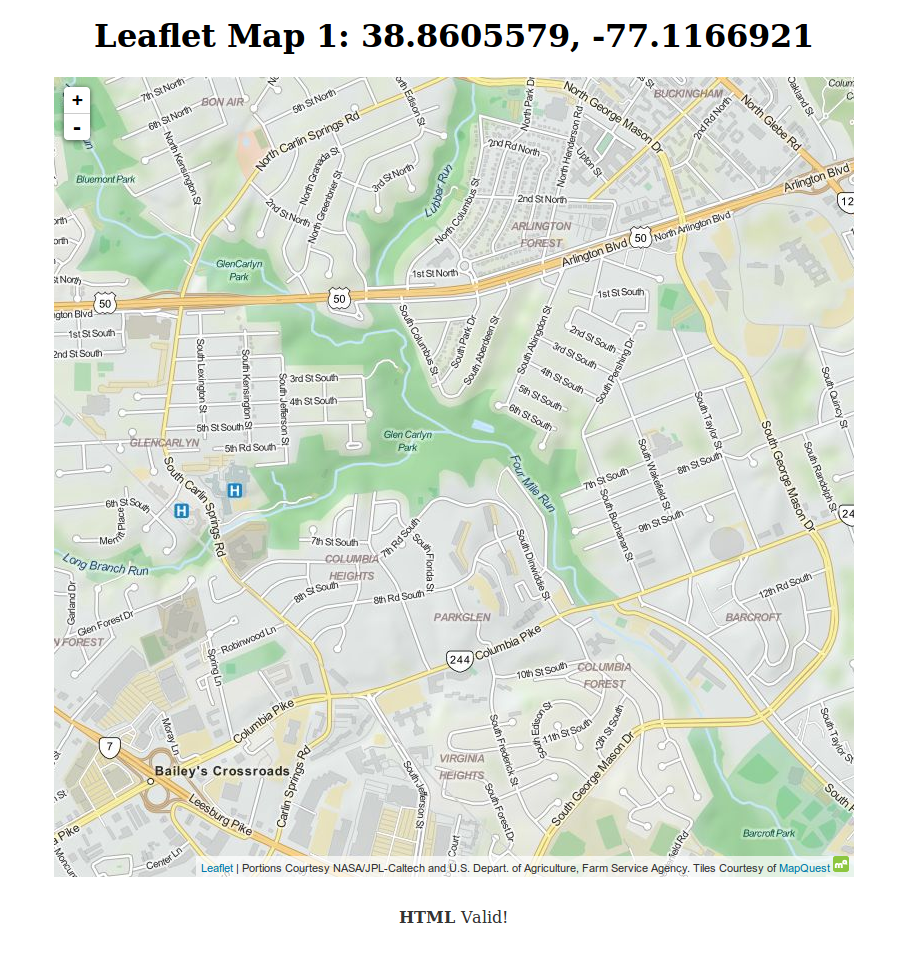
A few changes, and it uses my tilestache server instead:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Leaflet Map 2: Arlington Civic Associations</title>
<style type="text/css">
@import url(http://cdn.leafletjs.com/leaflet/v0.7.7/leaflet.css);
h1 {
text-align: center;
}
#map {
height: 900px;
width: 900px;
margin-left: auto;
margin-right: auto;
}
footer {
text-align: center;
margin-top: 30px;
}
footer a, footer a:visited {
text-decoration: none;
color: #333;
}
</style>
<script src="http://cdn.leafletjs.com/leaflet/v0.7.7/leaflet.js"></script>
</head>
<body>
<h1>Leaflet Map 2: Arlington Civic Associations</h1>
<div id="map"></div>
<script>
var map = L.map('map').setView([38.8800, -77.1050], 13);
L.tileLayer('http://host.machine:8080/ex/{z}/{x}/{y}.png', {
attribution: 'Map Arlington',
maxZoom: 16,
}).addTo(map);
</script>
<footer>
<a href="http://validator.w3.org/check/referer">
<strong> HTML </strong> Valid! </a>
</footer>
</body>
</html>
Which looks like this:
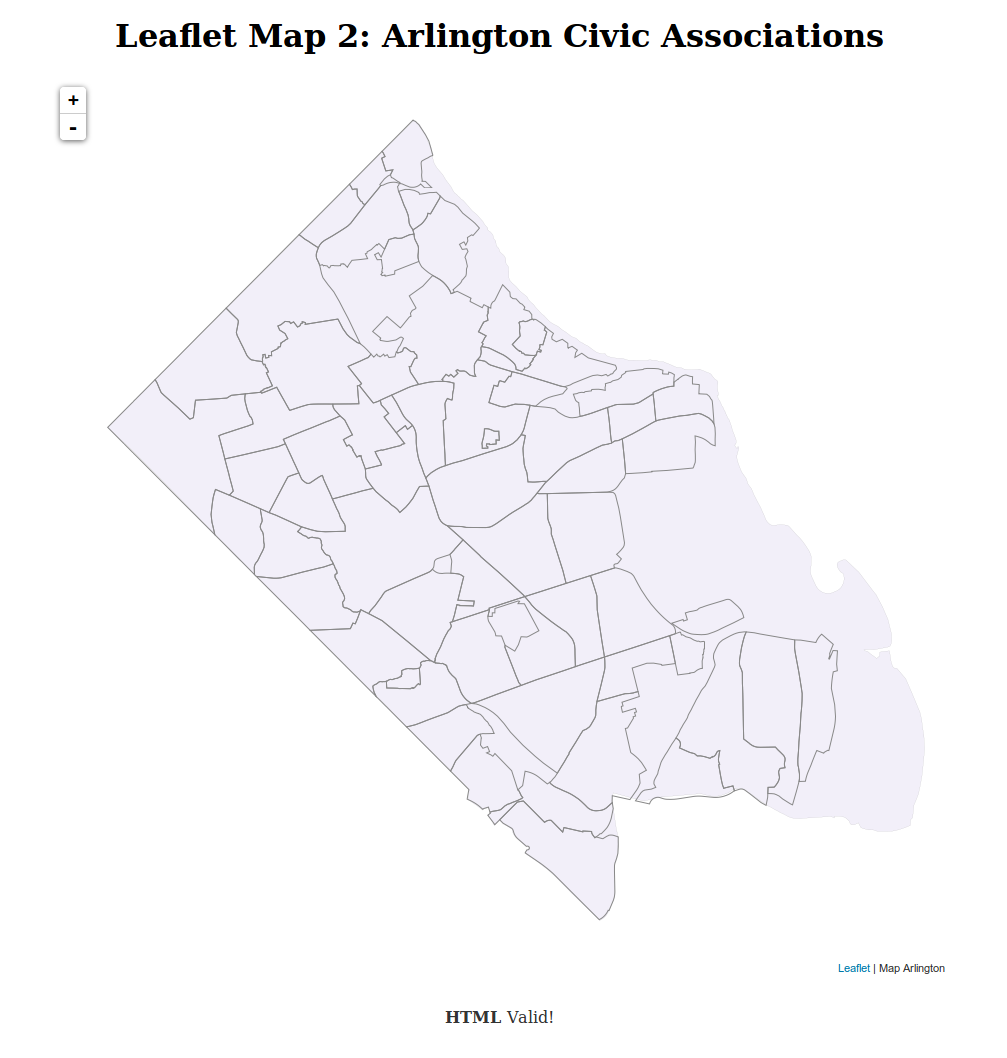